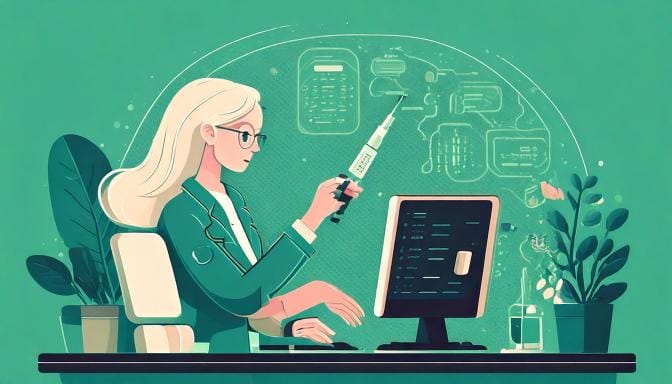
Understanding Dependency Injection in Kotlin Spring Boot: A Practical Guide
Explore how to implement dependency injection effectively in Kotlin Spring Boot projects. Learn about constructor injection, property injection, and method injection with real-world examples and best practices for creating maintainable applications.
Dependency Injection (DI) is a fundamental concept in Spring Boot that helps create loosely coupled, maintainable applications. In this guide, we'll explore how to implement DI effectively in your Kotlin Spring Boot projects with practical examples.
Key Takeaways
- Learn the three main types of dependency injection in Spring Boot
- Understand how to use Kotlin-specific features with Spring DI
- Master best practices for testing and maintaining DI-based code
- Explore common pitfalls and their solutions
What is Dependency Injection?
Dependency Injection is a design pattern where components receive their dependencies from an external source rather than creating them internally. In Spring Boot, this is handled automatically by the Spring container.
Let's look at a simple example. Consider a notification service that needs to send emails:
// Without Dependency Injection (Not Recommended)
class NotificationService {
private val emailSender = EmailSender() // Tight coupling!
fun notifyUser(userId: String, message: String) {
emailSender.sendEmail(userId, message)
}
}
Here's the same service using dependency injection:
// With Dependency Injection (Recommended)
@Service
class NotificationService(
private val emailSender: EmailSender // Injected by Spring
) {
fun notifyUser(userId: String, message: String) {
emailSender.sendEmail(userId, message)
}
}
Types of Dependency Injection in Spring Boot
1. Constructor Injection (Recommended)
Constructor injection is the preferred method in Kotlin Spring Boot applications. It ensures that required dependencies are available during object creation:
@Service
class OrderService(
private val repository: OrderRepository,
private val paymentService: PaymentService
) {
fun processOrder(order: Order): OrderResult {
val savedOrder = repository.save(order)
val paymentResult = paymentService.process(order.payment)
return OrderResult(savedOrder, paymentResult)
}
}
2. Property Injection
While not recommended for required dependencies, property injection can be useful for optional dependencies:
@Service
class MetricsService {
@Autowired
private lateinit var optionalAnalytics: AnalyticsService?
fun recordMetric(metric: Metric) {
// Core functionality
metric.process()
// Optional analytics
optionalAnalytics?.record(metric)
}
}
3. Method Injection
Method injection is less common but useful when you need to inject dependencies based on method parameters:
@Service
class ConfigurationService {
@Autowired
private lateinit var context: ApplicationContext
fun <T> loadConfig(configClass: Class<T>): T {
return context.getBean(configClass)
}
}
Kotlin-Specific Features with Spring DI
Using Nullability
Kotlin's null safety features work well with Spring's dependency injection:
@Service
class UserService(
private val repository: UserRepository,
private val notifier: NotificationService? = null // Optional dependency
) {
fun createUser(user: User) {
val savedUser = repository.save(user)
notifier?.sendWelcomeMessage(savedUser) // Safe call
}
}
Using by lazy
Kotlin's lazy
delegation can be useful for dependencies that are expensive to create:
@Service
class CacheService(
private val cacheManager: CacheManager
) {
private val expensiveCache by lazy {
cacheManager.createCache("expensive-cache", ComplexConfig())
}
fun getData(key: String): Any? = expensiveCache.get(key)
}
Testing with Dependency Injection
Spring Boot makes it easy to test components that use dependency injection:
@SpringBootTest
class NotificationServiceTest {
@MockBean
private lateinit var emailSender: EmailSender
@Autowired
private lateinit var notificationService: NotificationService
@Test
fun `should send notification successfully`() {
// Given
val userId = "user123"
val message = "Hello!"
// When
notificationService.notifyUser(userId, message)
// Then
verify(emailSender).sendEmail(userId, message)
}
}
Common Pitfalls and Best Practices
- Circular Dependencies
Avoid creating circular dependencies between components:
// DON'T DO THIS
@Service
class ServiceA(private val serviceB: ServiceB)
@Service
class ServiceB(private val serviceA: ServiceA) // Circular dependency!
- Constructor Injection vs. Field Injection
Prefer constructor injection for required dependencies:
// GOOD
@Service
class UserService(
private val repository: UserRepository
)
// AVOID
@Service
class UserService {
@Autowired
private lateinit var repository: UserRepository
}
- Component Scanning
Use appropriate component scanning to ensure Spring finds your components:
@SpringBootApplication
@ComponentScan(basePackages = ["com.yourapp"])
class YourApplication
fun main(args: Array<String>) {
runApplication<YourApplication>(*args)
}
Conclusion
Dependency Injection is a powerful feature in Spring Boot that, when used correctly, leads to more maintainable and testable code. By following Kotlin best practices and Spring Boot conventions, you can create robust applications that are easy to develop and maintain.
Remember to:
- Use constructor injection for required dependencies
- Leverage Kotlin's null safety features for optional dependencies
- Write tests that take advantage of Spring's testing support
- Avoid common pitfalls like circular dependencies