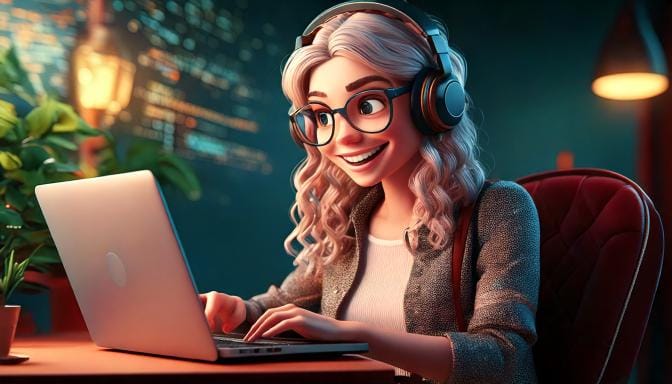
Why You Should Disable CSRF Protection for REST APIs in Spring Boot (And How to Do It Right)
Learn why CSRF protection isn't necessary for most REST APIs and how to properly disable it in Spring Boot while maintaining security. Includes code examples and best practices
Why You Should Disable CSRF Protection for REST APIs in Spring Boot (And How to Do It Right)
Cross-Site Request Forgery (CSRF) protection is a crucial security measure for traditional web applications, but when it comes to REST APIs, it's often unnecessary and can even complicate your architecture. Let's understand why and how to properly disable it in Spring Boot.
Understanding the Context: Why CSRF Exists
CSRF attacks work by tricking a user's browser into making unwanted requests to a server where the user is already authenticated. This attack is particularly dangerous for traditional web applications because:
- They rely on session cookies for authentication
- Browsers automatically include cookies in requests
- Users directly interact with the web interface
Why REST APIs Are Different
Modern REST APIs typically use different authentication mechanisms that make them naturally resistant to CSRF attacks. Here's why:
- Token-based Authentication: REST APIs commonly use JWT or API keys sent in the Authorization header. Unlike cookies, these tokens aren't automatically included in requests by browsers.
- Stateless Nature: REST APIs don't rely on sessions, eliminating the primary vector that CSRF attacks exploit.
- Cross-Origin Requests: REST APIs are typically consumed by frontend applications that explicitly handle authentication, not through direct browser interactions.
How to Properly Disable CSRF in Spring Boot
Here's how to disable CSRF protection for your REST API:
@Configuration
@EnableWebSecurity
class SecurityConfig {
@Bean
fun filterChain(http: HttpSecurity): SecurityFilterChain {
return http.csrf {
it.disable()
}.build()
}
}
Best Practices When Disabling CSRF
While disabling CSRF for REST APIs is generally safe, you should implement these complementary security measures:
- Use HTTPS: Always enforce HTTPS to prevent token interception.
- Implement CORS Properly: Configure strict CORS policies to control which domains can access your API.
- Set Secure Headers: Include security headers like Content-Security-Policy.
@Configuration
@EnableWebSecurity
class SecurityConfig {
@Bean
fun filterChain(http: HttpSecurity): SecurityFilterChain {
return http.csrf {
it.disable()
}.build()
}
}
When You Might Still Want CSRF Protection
There are scenarios where you might want to keep CSRF protection:
- Your API uses cookie-based authentication
- Your API is directly accessed by browser-based forms
- You're building a hybrid application that mixes traditional web pages with REST endpoints
Conclusion
Disabling CSRF for REST APIs is not just a simplification—it's often the right architectural choice. By understanding the context and implementing proper security alternatives, you can build secure APIs without unnecessary complexity.
Remember: Security is about implementing the right protections for your specific use case, not about enabling every possible security measure regardless of context