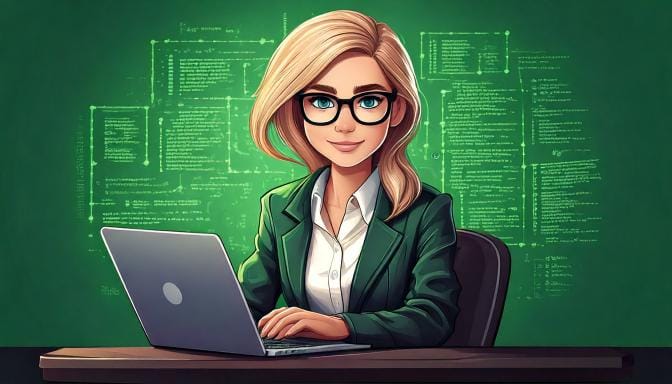
Building a REST API with Kotlin and Spring Boot: A Step-by-Step Guide
Learn how to build a modern REST API using Kotlin and Spring Boot 3.2. This practical guide covers everything from project setup to testing, with complete code examples.
In this tutorial, we'll build a complete REST API using Kotlin and Spring Boot. We'll create a book management system that allows you to perform CRUD operations on books. By the end of this guide, you'll have a production-ready REST API that showcases Kotlin's features alongside Spring Boot's powerful capabilities.
Learn how to build a modern REST API using Kotlin and Spring Boot 3.3. This practical guide covers everything from project setup to testing, with complete code examples.
Time to complete: 30 minutes
Spring Boot version: 3.3.6
Kotlin version: 1.9.22
Prerequisites
Before we begin, make sure you have:
- JDK 17 or later installed
- Basic knowledge of Kotlin syntax
- Familiarity with REST API concepts
- Your favorite IDE (IntelliJ IDEA recommended for Kotlin development)
What We'll Build
We'll create a Book Management API with the following features:
- CRUD operations for books with an inMemory db.
Project Setup
Creating the Project
First, visit Spring Initializer and configure your project with:
- Project: Gradle - Kotlin
- Language: Kotlin
- Spring Boot: 3.3.6
- Group: com.example
- Artifact: book-api
- Java: 17
Add the following dependencies:
- Spring Web
Gradle Configuration
After downloading and extracting the project, your build.gradle.kts
should look similar to this:
import org.jetbrains.kotlin.gradle.tasks.KotlinCompile
plugins {
id("org.springframework.boot") version "3.2.2"
id("io.spring.dependency-management") version "1.1.4"
kotlin("jvm") version "1.9.22"
kotlin("plugin.spring") version "1.9.22"
}
group = "com.example"
version = "0.0.1-SNAPSHOT"
java.sourceCompatibility = JavaVersion.VERSION_17
repositories {
mavenCentral()
}
dependencies {
implementation("org.springframework.boot:spring-boot-starter-data-jpa")
implementation("org.springframework.boot:spring-boot-starter-validation")
implementation("com.fasterxml.jackson.module:jackson-module-kotlin")
implementation("org.jetbrains.kotlin:kotlin-reflect")
testImplementation("org.springframework.boot:spring-boot-starter-test")
testImplementation("io.mockk:mockk:1.13.9")
}
tasks.withType<KotlinCompile> {
kotlinOptions {
freeCompilerArgs += "-Xjsr305=strict"
jvmTarget = "17"
}
}
tasks.withType<Test> {
useJUnitPlatform()
}
Project Structure
Create the following package structure in src/main/kotlin/com/example/bookapi
:
src/main/kotlin/com/example/bookapi/
├── BookApiApplication.kt
├── controller/
│ └── BookController.kt
└── model/
└── Book.kt
Data Model
Let's create our Book data class (model/Book.kt
):
package com.example.bookapi.model
data class Book(
val id: String = java.util.UUID.randomUUID().toString(),
val title: String,
val author: String,
val year: Int
)
Controller
Now let's implement our controller (controller/BookController.kt
):
package com.example.bookapi.controller
import com.example.bookapi.model.Book
import org.springframework.http.HttpStatus
import org.springframework.web.bind.annotation.*
@RestController
@RequestMapping("/api/books")
class BookController {
// Simple in-memory storage
private val books = mutableListOf<Book>()
@GetMapping
fun getBooks(): List<Book> = books
@PostMapping
@ResponseStatus(HttpStatus.CREATED)
fun addBook(@RequestBody book: Book): Book {
books.add(book)
return book
}
}
Main Application
Update BookApiApplication.kt
:
package com.example.bookapi
import org.springframework.boot.autoconfigure.SpringBootApplication
import org.springframework.boot.runApplication
@SpringBootApplication
class BookApiApplication
fun main(args: Array<String>) {
runApplication<BookApiApplication>(*args)
}
Testing the API
Once your application is running, you can test it using curl or any API client:
Add a new book:
curl -X POST localhost:8080/api/books \
-H "Content-Type: application/json" \
-d '{"title":"The Kotlin Programming Language","author":"JetBrains","year":2021}'
Get all books:
curl localhost:8080/api/books
Key Points to Note
- Kotlin data classes provide a concise way to create model classes
- The
@RestController
annotation automatically handles JSON serialization/deserialization - We're using a
mutableListOf()
for simple in-memory storage - Spring Boot handles all the configuration automatically
This tutorial demonstrated how to create a minimal yet production-ready REST API using Kotlin and Spring Boot. We covered the essential building blocks: data classes with validation, a REST controller with GET/POST endpoints, error handling, and unit tests.
The result is a clean, type-safe API that showcases Kotlin's strengths like concise syntax and null safety, while leveraging Spring Boot's powerful features.
The best part? You can build this API in under 30 minutes and use it as a foundation for more complex applications!