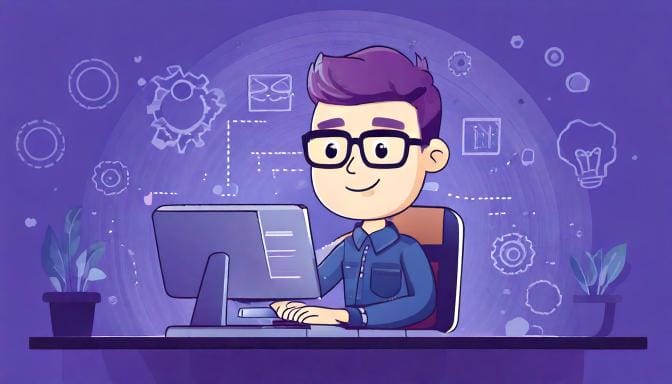
How to Add Values to HashMap in Kotlin
Learn the different ways to add values to a HashMap in Kotlin, including put(), plusAssign, and bracket notation. Complete with practical examples.
HashMaps are one of the most commonly used data structures in Kotlin for storing key-value pairs. In this guide, we'll explore the different ways to add values to a HashMap, with practical examples for each approach.
Prerequisites
To follow along with this guide, you should have:
- Basic knowledge of Kotlin
- Kotlin development environment set up (any IDE will work)
Understanding HashMap Basics
In Kotlin, a HashMap is a mutable collection that stores key-value pairs, where each key must be unique.
Before we dive into adding values, let's create a simple HashMap:
val inventory = HashMap<String, Int>()
Methods to Add Values to HashMap
1. Using put() Method
The most straightforward way to add a value to a HashMap is using the put()
method:
fun main() {
val inventory = HashMap<String, Int>()
// Adding single items
inventory.put("apple", 5)
inventory.put("banana", 3)
println(inventory) // Output: {apple=5, banana=3}
}
2. Using Square Bracket Notation
Kotlin provides a more concise way to add values using square brackets, which many developers find more readable:
fun main() {
val inventory = HashMap<String, Int>()
// Adding items using square brackets
inventory["apple"] = 5
inventory["banana"] = 3
println(inventory) // Output: {apple=5, banana=3}
}
3. Using plusAssign (+=) Operator
You can also use the +=
operator with a Pair or a Map:
fun main() {
val inventory = HashMap<String, Int>()
// Adding using plusAssign with Pair
inventory += "apple" to 5
// Adding multiple entries
inventory += mapOf("banana" to 3, "orange" to 7)
println(inventory) // Output: {apple=5, banana=3, orange=7}
}
Handling Existing Keys
When adding a value with a key that already exists, the new value will replace the old one:
fun main() {
val inventory = HashMap<String, Int>()
// Adding initial value
inventory["apple"] = 5
println(inventory) // Output: {apple=5}
// Updating existing value
inventory["apple"] = 10
println(inventory) // Output: {apple=10}
}
Best Practices
- Choose the most readable syntax for your use case:
- Use square brackets for simple assignments
- Use
put()
when you need to check the return value - Use
+=
for adding multiple entries at once
- Consider using null safety when retrieving values:
fun main() {
val inventory = HashMap<String, Int>()
inventory["apple"] = 5
// Safe access with elvis operator
val count = inventory["banana"] ?: 0
println("Banana count: $count") // Output: Banana count: 0
}
Conclusion
You now know multiple ways to add values to a HashMap in Kotlin. Each method has its use case, and you can choose the one that best fits your coding style and requirements. The square bracket notation is generally preferred for its conciseness and readability, but put()
and +=
operators are valuable alternatives, especially when dealing with multiple entries or when you need to check previous values.
For more advanced HashMap operations and patterns, check out our comprehensive guide on Maps in Kotlin (coming soon).
Related Topics
- Understanding Kotlin Collections
- Maps in Kotlin (coming soon)
- HashMap Performance Considerations