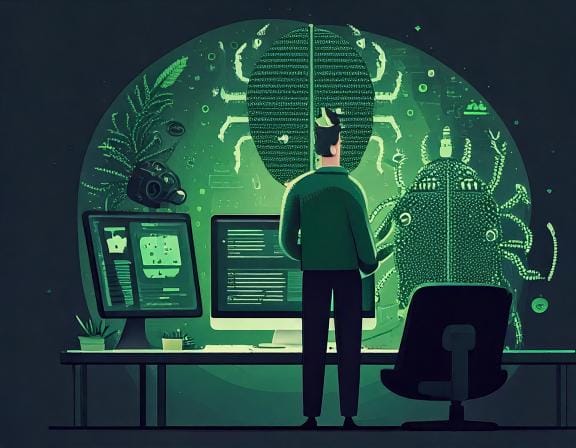
How to Fix "Cannot Convert String to JSON Object" Error in Kotlin Spring Boot
Learn how to properly handle JSON string conversion errors in Kotlin Spring Boot applications with practical solutions and best practices
When working with JSON in Spring Boot applications, you might encounter the error "Cannot Convert java.lang.String to JSON Object". This error typically occurs when there's a mismatch between your JSON string format and how you're trying to parse it. In this guide, we'll explore why this happens and show you several ways to fix it.
Understanding the Problem
This error usually appears in one of these scenarios:
- Receiving JSON data through a REST endpoint
- Reading JSON from a file or external source
- Converting between String and JSONObject types
Let's look at a typical example where this error occurs:
@RestController
class UserController {
@PostMapping("/users")
fun createUser(@RequestBody userJson: String): ResponseEntity<User> {
// This might throw the conversion error
val user = ObjectMapper().readValue(userJson, User::class.java)
return ResponseEntity.ok(user)
}
}
Solution 1: Using Proper Request Body Mapping
The most straightforward solution is to let Spring Boot handle the JSON conversion automatically by defining a data class:
// Define your data structure
data class User(
val name: String,
val email: String,
val age: Int
)
@RestController
class UserController {
@PostMapping("/users")
fun createUser(@RequestBody user: User): ResponseEntity<User> {
// Spring Boot automatically converts the JSON to User object
return ResponseEntity.ok(user)
}
}
This approach lets Spring's built-in JSON conversion handle everything for you, preventing the "Cannot Convert" error entirely.
Solution 2: Manual JSON String Conversion
If you need to work with raw JSON strings, here's how to properly convert them:
import com.fasterxml.jackson.module.kotlin.jacksonObjectMapper
import com.fasterxml.jackson.module.kotlin.readValue
class JsonService {
private val mapper = jacksonObjectMapper()
fun convertJsonString(jsonString: String): User {
return try {
mapper.readValue<User>(jsonString)
} catch (e: Exception) {
throw JsonParseException("Invalid JSON format: ${e.message}")
}
}
}
Note that we're using jacksonObjectMapper()
from the Kotlin module, which provides better Kotlin support than the standard ObjectMapper
.
Solution 3: Working with Dynamic JSON
Sometimes you need to handle dynamic JSON where the structure isn't known at compile time:
import com.fasterxml.jackson.databind.JsonNode
class DynamicJsonService {
private val mapper = jacksonObjectMapper()
fun processDynamicJson(jsonString: String): JsonNode {
return try {
mapper.readTree(jsonString)
} catch (e: Exception) {
throw JsonParseException("Failed to parse JSON: ${e.message}")
}
}
fun extractValue(jsonNode: JsonNode, path: String): String? {
return jsonNode.at(path).asText(null)
}
}
// Usage example
fun main() {
val service = DynamicJsonService()
val json = """{"user": {"name": "John", "details": {"age": 30}}}"""
val jsonNode = service.processDynamicJson(json)
val name = service.extractValue(jsonNode, "/user/name")
println(name) // Output: John
}
Best Practices for JSON Handling
- Always validate your JSON input:
fun isValidJson(jsonString: String): Boolean {
return try {
jacksonObjectMapper().readTree(jsonString)
true
} catch (e: Exception) {
false
}
}
- Use proper exception handling:
@RestController
class UserController {
@PostMapping("/users")
fun createUser(@RequestBody userJson: String): ResponseEntity<*> {
return try {
val user = jacksonObjectMapper().readValue<User>(userJson)
ResponseEntity.ok(user)
} catch (e: Exception) {
ResponseEntity
.status(HttpStatus.BAD_REQUEST)
.body("Invalid JSON format: ${e.message}")
}
}
}
- Configure Jackson for Kotlin:
@Configuration
class JacksonConfig {
@Bean
fun objectMapper(): ObjectMapper {
return jacksonObjectMapper()
.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false)
.registerModule(JavaTimeModule())
}
}
Common Pitfalls to Avoid
- Don't mix different JSON libraries in the same project. Stick to Jackson (Spring Boot's default) unless you have a specific reason to use another library.
- Remember that JSON property names are case-sensitive:
// This will work
val validJson = """{"name": "John", "age": 30}"""
// This will fail
val invalidJson = """{"Name": "John", "Age": 30}"""
data class User(
val name: String, // Notice lowercase property names
val age: Int
)
- Be careful with nullable properties:
// Define nullable fields properly
data class User(
val name: String,
val email: String?, // Nullable field
val age: Int
)
Conclusion
The "Cannot Convert String to JSON Object" error is usually easy to fix once you understand what's causing it. By following the solutions and best practices outlined in this guide, you can handle JSON conversion properly in your Kotlin Spring Boot applications.
Related Topics
- Understanding Jackson ObjectMapper in Kotlin
- Working with REST APIs in Spring Boot
- JSON Validation Techniques
- Error Handling Best Practices in Spring Boot