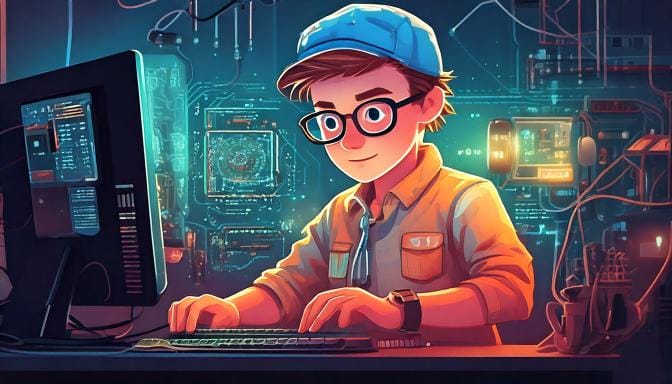
Implementing Retry Mechanisms in Kotlin Spring Boot Apps
Learn how to implement resilient retry mechanisms in Kotlin Spring Boot applications using Spring Retry, including exponential backoff, conditional retries, and best practices for handling transient failures in microservices
Introduction
In distributed systems and microservices architectures, temporary failures are inevitable. Network hiccups, service unavailability, or temporary database locks can cause operations to fail. Implementing retry mechanisms is a crucial resilience pattern that can help your application handle these transient failures gracefully.
In this tutorial, you'll learn how to implement retry mechanisms in a Kotlin Spring Boot application. You'll explore different retry strategies using Spring Retry and learn how to handle various failure scenarios effectively.
Prerequisites
To follow this tutorial, you will need:
- Basic knowledge of Kotlin and Spring Boot
- JDK 17 or later installed on your system
- Your favorite IDE (IntelliJ IDEA recommended for Kotlin development)
- Gradle or Maven build tool
- Basic understanding of RESTful services
Step 1 — Setting Up the Project
First, create a new Spring Boot project with Kotlin. You can use Spring Initializer (start.spring.io) with the following dependencies:
- Spring Web
- Spring Retry
- Spring AOP
Your build.gradle.kts
should include these dependencies:
dependencies {
implementation("org.springframework.boot:spring-boot-starter-web")
implementation("org.springframework.retry:spring-retry")
implementation("org.springframework.boot:spring-boot-starter-aop")
implementation("com.fasterxml.jackson.module:jackson-module-kotlin")
implementation("org.jetbrains.kotlin:kotlin-reflect")
}
Step 2 — Enabling Retry Support
To enable retry support in your application, add the @EnableRetry
annotation to your main application class:
import org.springframework.retry.annotation.EnableRetry
import org.springframework.boot.autoconfigure.SpringBootApplication
import org.springframework.boot.runApplication
@EnableRetry
@SpringBootApplication
class RetryDemoApplication
fun main(args: Array<String>) {
runApplication<RetryDemoApplication>(*args)
}
Step 3 — Creating a Basic Service with Retry
Let's create a simple service that simulates an external API call with potential failures:
import org.springframework.retry.annotation.Backoff
import org.springframework.retry.annotation.Recover
import org.springframework.retry.annotation.Retryable
import org.springframework.stereotype.Service
import java.net.SocketTimeoutException
@Service
class ExternalServiceClient {
@Retryable(
value = [SocketTimeoutException::class],
maxAttempts = 3,
backoff = Backoff(delay = 1000)
)
fun fetchData(id: String): String {
// Simulate random failure
if (Math.random() < 0.7) {
throw SocketTimeoutException("Connection timed out")
}
return "Data for ID: $id"
}
@Recover
fun recover(e: SocketTimeoutException, id: String): String {
return "Fallback data for ID: $id"
}
}
This service includes:
@Retryable
annotation specifying which exceptions to retry- Maximum number of attempts (3 in this case)
- Backoff delay between retries (1 second)
- A recovery method using
@Recover
for when all retries fail
Step 4 — Implementing Different Retry Strategies
Exponential Backoff
For more sophisticated retry scenarios, you might want to use exponential backoff.
Understanding Exponential Backoff
When a service fails, immediately retrying might not be the best strategy. If the service is down due to high load, bombarding it with immediate retries could make the situation worse. This is where exponential backoff comes in.
Exponential backoff is a retry strategy where you progressively increase the waiting time between retry attempts. Each retry attempt waits longer than the previous one. For example:
- First retry: Wait 1 second
- Second retry: Wait 2 seconds
- Third retry: Wait 4 seconds
- Fourth retry: Wait 8 seconds
Think of it like giving someone increasingly longer breaks when they're overwhelmed. Instead of constantly asking "Is it fixed yet? Is it fixed yet?", you give the service more breathing room between each attempt.
Why Use Exponential Backoff?
- Prevents System Overload: If a service is struggling with high load, exponential backoff naturally spreads out retry attempts, giving the system time to recover.
- Network Issues: Temporary network problems often resolve themselves within a few seconds. Exponential backoff helps you wait out these transient issues without wasting resources.
- Resource Efficiency: Instead of wasting resources on rapid-fire retries that are likely to fail, your application conserves resources by waiting longer between attempts.
Here's a practical example: Imagine your application calling a payment service that's experiencing issues. With exponential backoff:
@Service
class AdvancedRetryService {
@Retryable(
value = [ServiceException::class],
maxAttempts = 4,
backoff = Backoff(
delay = 1000,
multiplier = 2.0,
maxDelay = 4000
)
)
fun performOperation(): String {
// Service logic here
throw ServiceException("Service temporarily unavailable")
}
}
If the payment service is overloaded, your first retry happens after 1 second, then 2 seconds, then 4 seconds – giving it an increasingly better chance of success with each attempt.
Conditional Retry
Sometimes you want to retry only under specific conditions:
@Service
class ConditionalRetryService {
@Retryable(
value = [ServiceException::class],
maxAttempts = 3,
include = [RetryableException::class]
)
fun processData(data: String): Result {
if (isTransientError()) {
throw RetryableException("Temporary error")
}
if (isPermanentError()) {
throw PermanentException("Permanent error")
}
return Result(data)
}
}
Step 5 — Adding a REST Controller
Create a REST controller to expose your retry-enabled service:
import org.springframework.web.bind.annotation.*
@RestController
@RequestMapping("/api")
class RetryController(
private val externalServiceClient: ExternalServiceClient,
private val advancedRetryService: AdvancedRetryService
) {
@GetMapping("/data/{id}")
fun getData(@PathVariable id: String): String {
return externalServiceClient.fetchData(id)
}
@PostMapping("/process")
fun processData(): String {
return advancedRetryService.performOperation()
}
}
Step 6 — Implementing Custom Retry Templates
For more complex scenarios, you might want to use programmatic retry using RetryTemplate:
import org.springframework.retry.support.RetryTemplate
import org.springframework.context.annotation.Bean
import org.springframework.context.annotation.Configuration
@Configuration
class RetryConfig {
@Bean
fun retryTemplate(): RetryTemplate {
return RetryTemplate().apply {
setRetryPolicy(SimpleRetryPolicy(3))
setBackOffPolicy(ExponentialBackOffPolicy().apply {
initialInterval = 1000
multiplier = 2.0
maxInterval = 5000
})
}
}
}
@Service
class TemplateRetryService(private val retryTemplate: RetryTemplate) {
fun executeWithRetry(operation: () -> String): String {
return retryTemplate.execute<String, Exception> {
operation()
}
}
}
Step 7 — Monitoring and Logging Retries
Add logging to track retry attempts:
import org.slf4j.LoggerFactory
@Service
class MonitoredRetryService {
private val logger = LoggerFactory.getLogger(javaClass)
@Retryable(
value = [ServiceException::class],
maxAttempts = 3,
listeners = ["retryListener"]
)
fun execute() {
logger.info("Attempting operation...")
throw ServiceException("Service unavailable")
}
}
@Component
class RetryListener : RetryListener {
private val logger = LoggerFactory.getLogger(javaClass)
override fun <T, E : Throwable> onError(
context: RetryContext,
callback: RetryCallback<T, E>,
throwable: Throwable
) {
logger.error("Retry attempt ${context.retryCount} failed", throwable)
}
}
Step 8 — Testing Retry Mechanisms
Create tests to verify your retry logic:
@SpringBootTest
class RetryServiceTest {
@Autowired
lateinit var externalServiceClient: ExternalServiceClient
@Test
fun `should retry and eventually succeed`() {
val result = externalServiceClient.fetchData("test-id")
assertNotNull(result)
}
@Test
fun `should use fallback after max retries`() {
// Force failure
val result = externalServiceClient.fetchData("failing-id")
assertTrue(result.startsWith("Fallback"))
}
}
Best Practices and Considerations
- Choose Appropriate Retry Intervals:
- Start with small intervals for quick operations
- Use exponential backoff for external service calls
- Set maximum retry attempts to prevent infinite loops
- Handle Different Types of Failures:
- Retry only for transient failures
- Fail fast for permanent errors
- Implement proper fallback mechanisms
- Monitor and Alert:
- Log retry attempts
- Track retry success/failure rates
- Set up alerts for excessive retries
- Circuit Breaker Integration:
- Consider combining retry with circuit breaker pattern
- Prevent cascade failures
- Implement proper fallback mechanisms
Conclusion
You've learned how to implement various retry mechanisms in a Kotlin Spring Boot application. From simple retries to complex patterns with exponential backoff and custom templates, you now have the tools to make your applications more resilient to transient failures.
The complete source code for this tutorial is available on GitHub [link to repository].
Next Steps
- Learn about Circuit Breakers to complement your retry mechanisms
- Explore Spring Cloud for distributed system patterns
- Implement metrics and monitoring for your retry mechanisms
Troubleshooting Common Issues
Issue 1: Retries Not Working
- Ensure
@EnableRetry
is present - Check AOP dependencies
- Verify method is public and called from another bean
Issue 2: Infinite Retries
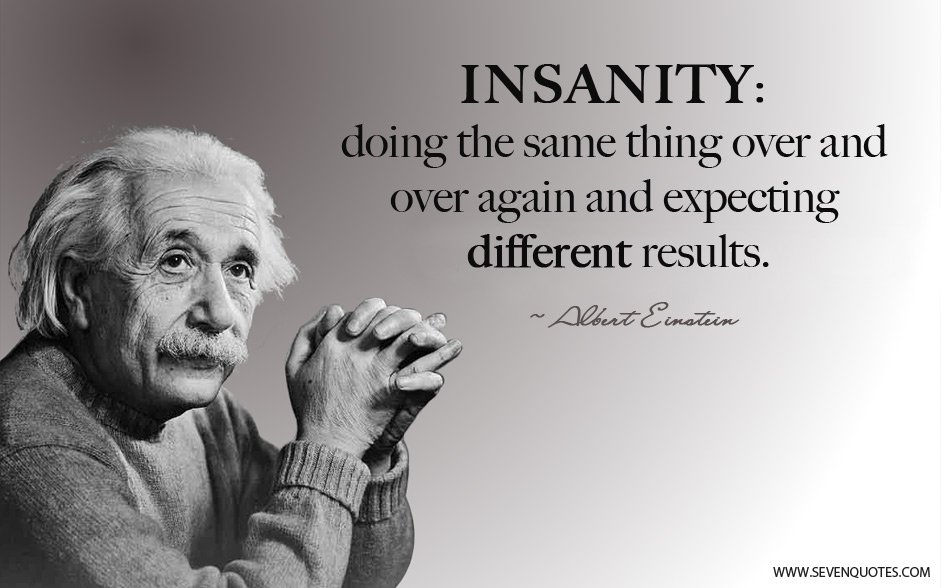
- Always set maxAttempts
- Implement proper recovery methods
- Use appropriate exception types
Issue 3: Performance Impact
- Monitor retry latency
- Adjust backoff parameters
- Consider async operations for long-running tasks