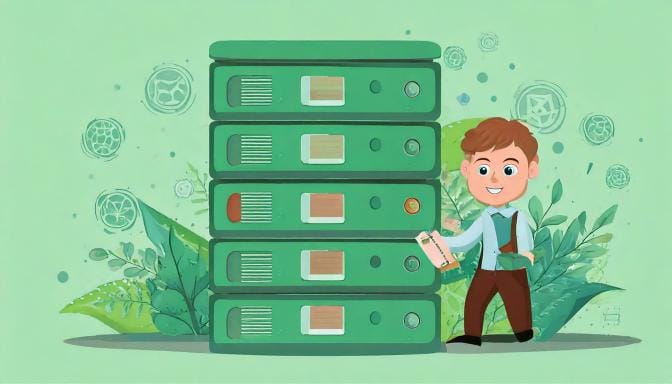
Repository Pattern in Spring Boot with Kotlin
Master the Repository pattern in Spring Boot using Kotlin. Learn how to structure your data access layer, implement custom repositories, and follow best practices for clean, maintainable code. Includes practical examples and common pitfalls to avoid.
The Repository pattern is a fundamental design pattern that abstracts your data access layer, making your Spring Boot applications more maintainable and testable. In this guide, we'll explore how to implement it effectively using Kotlin.
If you're new to Spring Boot with Kotlin, check our Getting Started guide first.
Key Takeaways
- Learn how to implement the Repository pattern in Spring Boot with Kotlin
- Understand the benefits of using Spring Data JPA repositories
- Master custom repository implementations
- Discover best practices for testing repositories
- Handle common edge cases and errors effectively
What is the Repository Pattern?
The Repository pattern acts as an abstraction layer between your business logic and data access layer. It provides a more object-oriented view of the persistence layer, encapsulating the data access logic and allowing the rest of the application to be persistence-ignorant.
Basic Implementation
Let's start with a simple example. Consider a blog application with posts:
@Entity
data class BlogPost(
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
val id: Long = 0,
@Column(nullable = false)
val title: String,
@Column(nullable = false)
val content: String,
@Column(nullable = false)
val createdAt: LocalDateTime = LocalDateTime.now()
)
Using Spring Data JPA Repository
The simplest way to implement the Repository pattern is using Spring Data JPA:
interface BlogPostRepository : JpaRepository<BlogPost, Long> {
fun findByTitleContainingIgnoreCase(title: String): List<BlogPost>
fun findByCreatedAtAfter(date: LocalDateTime): List<BlogPost>
}
This gives you basic CRUD operations plus custom query methods.
Custom Repository Implementation
Sometimes you need more complex operations. Here's how to create a custom repository:
interface CustomBlogPostRepository {
fun findPopularPosts(minViews: Int): List<BlogPost>
fun findRelatedPosts(postId: Long): List<BlogPost>
}
@Repository
class CustomBlogPostRepositoryImpl(
private val entityManager: EntityManager
) : CustomBlogPostRepository {
override fun findPopularPosts(minViews: Int): List<BlogPost> {
val query = entityManager.createQuery("""
SELECT p FROM BlogPost p
WHERE p.views >= :minViews
ORDER BY p.views DESC
""".trimIndent(), BlogPost::class.java)
query.setParameter("minViews", minViews)
return query.resultList
}
override fun findRelatedPosts(postId: Long): List<BlogPost> {
// Implementation details
return emptyList()
}
}
// Combine both interfaces
interface BlogPostRepository : JpaRepository<BlogPost, Long>, CustomBlogPostRepository
Best Practices
1. Use Interface Segregation
Split large repositories into smaller, focused interfaces:
interface ReadOnlyBlogPostRepository {
fun findById(id: Long): BlogPost?
fun findAll(): List<BlogPost>
}
interface WritableBlogPostRepository {
fun save(blogPost: BlogPost): BlogPost
fun delete(blogPost: BlogPost)
}
interface BlogPostRepository :
ReadOnlyBlogPostRepository,
WritableBlogPostRepository
2. Implement Pagination
Always consider pagination for large datasets:
interface BlogPostRepository : JpaRepository<BlogPost, Long> {
fun findByAuthor(
author: String,
pageable: Pageable
): Page<BlogPost>
}
// Usage
@Service
class BlogPostService(
private val repository: BlogPostRepository
) {
fun getPostsByAuthor(
author: String,
page: Int,
size: Int
): Page<BlogPost> {
val pageable = PageRequest.of(page, size, Sort.by("createdAt").descending())
return repository.findByAuthor(author, pageable)
}
}
3. Handle Errors Gracefully
Use Kotlin's Result type for error handling:
interface BlogPostRepository {
fun findById(id: Long): Result<BlogPost>
fun save(blogPost: BlogPost): Result<BlogPost>
}
class BlogPostRepositoryImpl : BlogPostRepository {
override fun findById(id: Long): Result<BlogPost> = runCatching {
// Implementation
entityManager.find(BlogPost::class.java, id)
?: throw NoSuchElementException("Post not found")
}
}
Testing
Here's how to test your repositories effectively. For more details on autowiring in Spring Boot, see our complete guide to Spring Autowiring in Kotlin.
@DataJpaTest
class BlogPostRepositoryTest {
@Autowired
private lateinit var repository: BlogPostRepository
@Test
fun `should find posts by title`() {
// Given
val post = BlogPost(
title = "Test Post",
content = "Test Content"
)
repository.save(post)
// When
val found = repository.findByTitleContainingIgnoreCase("test")
// Then
assertThat(found).hasSize(1)
assertThat(found.first().title).isEqualTo("Test Post")
}
}
For more comprehensive testing strategies, see our guide to Unit Testing in Spring Boot.
Common Pitfalls to Avoid
- N+1 Query Problem
// Bad - causes N+1 queries
@Entity
data class BlogPost(
@OneToMany(fetch = FetchType.EAGER)
val comments: List<Comment>
)
// Good - use join fetch
interface BlogPostRepository : JpaRepository<BlogPost, Long> {
@Query("SELECT p FROM BlogPost p LEFT JOIN FETCH p.comments")
fun findAllWithComments(): List<BlogPost>
}
- Missing Indexing
@Entity
data class BlogPost(
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
val id: Long = 0,
@Column(nullable = false)
@Index(name = "idx_title") // Add index for frequently queried fields
val title: String
)
Conclusion
The Repository pattern, when implemented correctly in Spring Boot with Kotlin, provides a clean and maintainable way to handle data access. By following these best practices and avoiding common pitfalls, you can create robust and efficient repositories that serve your application's needs well.
Remember to:
- Use Spring Data JPA repositories for simple cases
- Implement custom repositories when needed
- Follow best practices for pagination and error handling
- Test your repositories thoroughly
- Be aware of and avoid common pitfalls