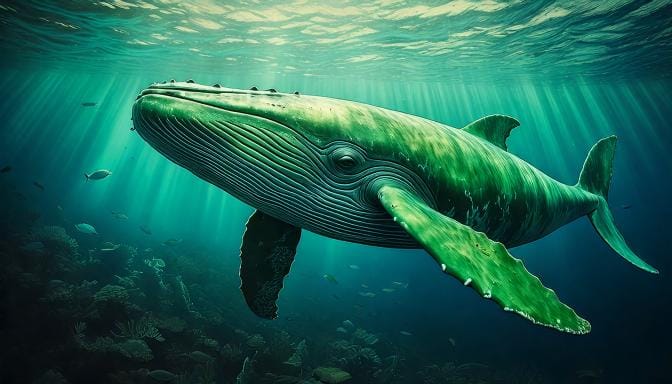
Implementing DeepSeek R1 in Spring Boot Applications: A Complete Guide
A practical guide to implementing DeepSeek R1 in Spring Boot applications using Spring AI. Learn how to configure and use this cost-effective AI solution with Spring Boot's powerful framework.
DeepSeek R1's cost-effective API and powerful capabilities make it an attractive choice for Spring Boot applications. In this guide, we'll walk through the process of integrating DeepSeek R1 with Spring Boot using Spring AI.
Key Takeaways
- Spring AI provides native support for DeepSeek integration
- Configuration can be done through application.properties
- DeepSeek offers significant cost savings at $0.55 per million tokens
- Implementation requires minimal setup with Spring Boot
Prerequisites
- Spring Boot project with Spring AI dependency
- DeepSeek API key
- Basic knowledge of Spring Boot
Implementation Steps
1. Add Dependencies
First, add the Spring AI dependency to your build.gradle.kts
:
dependencies {
implementation("org.springframework.ai:spring-ai-openai-spring-boot-starter:1.0.0-SNAPSHOT")
}
2. Configure DeepSeek
Add the following configuration to your application.properties
:
spring.ai.openai.chat.enabled=true
spring.ai.openai.chat.base-url=https://api.deepseek.com
spring.ai.openai.chat.api-key=your-api-key-here
spring.ai.openai.chat.options.model=deepseek-r1
3. Create a Service
Create a service class to handle DeepSeek interactions:
@Service
class DeepSeekAIService(
private val chatClient: ChatClient
) {
fun generateResponse(prompt: String): String {
val response = chatClient.call(prompt)
return response.content
}
fun generateStreamingResponse(prompt: String): Flow<String> = flow {
chatClient.stream(prompt).collect { response ->
emit(response.content)
}
}
}
4. Create a Controller
Set up a REST controller to expose the AI capabilities:
@RestController
@RequestMapping("/api/ai")
class DeepSeekController(
private val deepSeekAIService: DeepSeekAIService
) {
@PostMapping("/generate")
suspend fun generateResponse(
@RequestBody prompt: String
): String = deepSeekAIService.generateResponse(prompt)
@PostMapping("/generate/stream")
fun generateStreamingResponse(
@RequestBody prompt: String
): Flow<String> = deepSeekAIService.generateStreamingResponse(prompt)
}
Fine-tuning Configuration
Spring AI provides several configuration options for DeepSeek that you can set in application.properties:
Temperature Control
Control the creativity of responses by adjusting the temperature:
spring.ai.openai.chat.options.temperature=0.7
A higher temperature (closer to 1.0) makes responses more creative and diverse, while a lower temperature (closer to 0) makes them more focused and deterministic.
Token Limits
Set maximum tokens for responses:
spring.ai.openai.chat.options.maxTokens=2000
This controls the maximum length of the AI's responses. Adjust based on your needs while keeping in mind that longer responses consume more tokens.
Response Format
Enable JSON mode for structured responses:
spring.ai.openai.chat.options.responseFormat={"type": "json_object"}
This ensures responses are formatted as valid JSON, useful when you need structured data from the AI.
Additional Options
Other useful configuration options include:
# Penalize repeated content
spring.ai.openai.chat.options.frequencyPenalty=0.0
# Encourage new topics
spring.ai.openai.chat.options.presencePenalty=0.0
# Number of response choices
spring.ai.openai.chat.options.n=1
Using the AI Service
Here's how to use different features of the AI service:
Basic Text Generation
@Service
class ExampleService(
private val deepSeekAIService: DeepSeekAIService
) {
fun generateDocumentation(codeSnippet: String): String {
val prompt = """
Please analyze this code and generate documentation:
$codeSnippet
""".trimIndent()
return deepSeekAIService.generateResponse(prompt)
}
}
Streaming Responses
@Service
class StreamingExampleService(
private val deepSeekAIService: DeepSeekAIService
) {
fun streamCodeExplanation(code: String): Flow<String> {
val prompt = """
Explain this code step by step:
$code
""".trimIndent()
return deepSeekAIService.generateStreamingResponse(prompt)
}
}
Cost Considerations
DeepSeek R1's cost-effective API ($0.55 per million tokens) makes it an economical choice for production deployments. When designing your prompts and responses, consider:
- Keeping prompts concise but clear
- Setting appropriate token limits
- Using streaming for long responses
- Structuring prompts to get precise responses
Conclusion
DeepSeek R1's integration with Spring Boot through Spring AI provides a powerful and cost-effective way to add AI capabilities to your applications. The combination of Spring Boot's robust framework and DeepSeek's efficient API creates opportunities for building sophisticated AI-powered features.