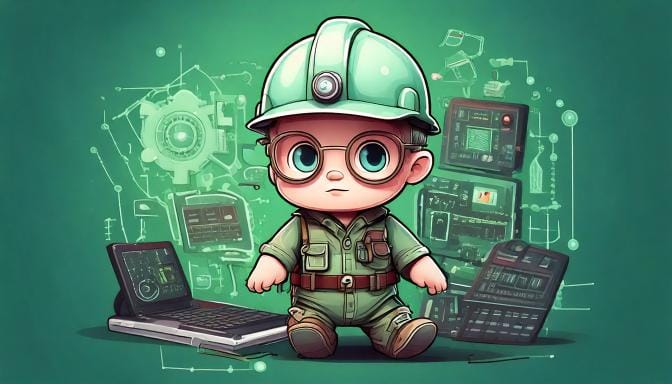
Setting Up Your First Kotlin Spring Boot Project
Get started with Kotlin Spring Boot development using this comprehensive guide. Learn how to set up a new project, understand the basic structure, and create your first endpoint with practical examples and best practices.
Creating your first Kotlin Spring Boot project doesn't have to be complicated. This guide will walk you through the process step by step, ensuring you have a solid foundation for building modern web applications.
Key Takeaways
- How to set up a new Kotlin Spring Boot project using Spring Initializr
- Understanding the basic project structure
- Essential dependencies for web development
- Running and testing your first application
Prerequisites
- JDK 17 or later installed
- An IDE (IntelliJ IDEA recommended)
- Basic Kotlin knowledge
Creating the Project
The easiest way to start is using Spring Initializr. You can either:
- Visit start.spring.io
- Use your IDE's Spring Initializr integration (recommended)
Let's create a simple web application:
// build.gradle.kts
plugins {
id("org.springframework.boot") version "3.2.2"
id("io.spring.dependency-management") version "1.1.4"
kotlin("jvm") version "1.9.22"
kotlin("plugin.spring") version "1.9.22"
}
group = "com.example"
version = "0.0.1-SNAPSHOT"
java {
sourceCompatibility = JavaVersion.VERSION_17
}
dependencies {
implementation("org.springframework.boot:spring-boot-starter-web")
implementation("com.fasterxml.jackson.module:jackson-module-kotlin")
implementation("org.jetbrains.kotlin:kotlin-reflect")
testImplementation("org.springframework.boot:spring-boot-starter-test")
}
Project Structure
After creation, your project will have this basic structure:
src
├── main
│ ├── kotlin
│ │ └── com
│ │ └── example
│ │ └── demo
│ │ ├── DemoApplication.kt
│ │ └── HelloController.kt
│ └── resources
│ └── application.properties
└── test
└── kotlin
└── com
└── example
└── demo
└── DemoApplicationTests.kt
Your First Controller
Let's create a simple controller to verify everything works:
// HelloController.kt
package com.example.demo
import org.springframework.web.bind.annotation.GetMapping
import org.springframework.web.bind.annotation.RestController
@RestController
class HelloController {
@GetMapping("/")
fun hello() = mapOf(
"message" to "Welcome to Kotlin Spring Boot!",
"timestamp" to System.currentTimeMillis()
)
}
Running the Application
Your main application file will look like this:
// DemoApplication.kt
package com.example.demo
import org.springframework.boot.autoconfigure.SpringBootApplication
import org.springframework.boot.runApplication
@SpringBootApplication
class DemoApplication
fun main(args: Array<String>) {
runApplication<DemoApplication>(*args)
}
Run the application and visit http://localhost:8080
to see your JSON response.
Testing
Spring Boot provides excellent testing support. Here's a basic test for our controller:
// DemoApplicationTests.kt
package com.example.demo
import org.junit.jupiter.api.Test
import org.springframework.beans.factory.annotation.Autowired
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc
import org.springframework.boot.test.context.SpringBootTest
import org.springframework.test.web.servlet.MockMvc
import org.springframework.test.web.servlet.get
@SpringBootTest
@AutoConfigureMockMvc
class DemoApplicationTests {
@Autowired
private lateinit var mockMvc: MockMvc
@Test
fun `should return welcome message`() {
mockMvc.get("/")
.andExpect {
status { isOk() }
jsonPath("$.message") { exists() }
jsonPath("$.timestamp") { exists() }
}
}
}
Common Issues and Solutions
- Port already in use
- Java version mismatch
- Ensure your JAVA_HOME points to JDK 17 or later
- Verify Gradle's Java compatibility settings
Solution: Change the port in application.properties
:
server.port=8081
Next Steps
- Add more dependencies based on your needs (JPA, Security, etc.)
- Configure application properties
- Set up database connections
- Implement more complex endpoints
Remember: Spring Boot with Kotlin provides a great developer experience, especially when you follow the conventions. Start simple and gradually add complexity as needed.