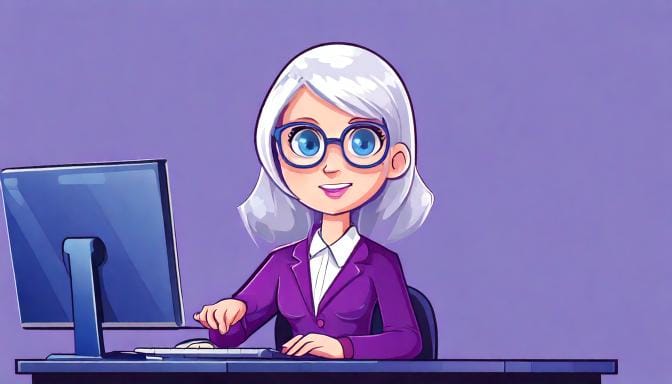
Understanding Kotlin's let Function: From Basics to Best Practices
Discover how to effectively use Kotlin's let function for null safety, transformations, and chaining operations. Learn best practices and common pitfalls with practical, real-world examples for writing cleaner, safer code.
The let
function is one of Kotlin's most versatile scope functions. While it might seem simple at first, understanding its nuances and proper usage patterns can significantly improve your code's readability and safety. In this guide, we'll explore everything you need to know about let
, from basic concepts to advanced patterns.
Understanding let
At its core, let
is a scope function that executes a block of code on an object and returns the result of that block. What makes let
special is how it handles the object context and its return value.
Here's a simple example:
val name: String? = "John"
val length = name?.let {
println("Processing name: $it")
it.length // Returns the length
}
In this example, let
does three important things:
- Provides a safe scope to work with the nullable
name
- Makes the name available as
it
within the block - Returns the length of the name (if name is not null)
Key Characteristics of let
The let
function has several distinctive features that set it apart from other scope functions:
- Context Object: Inside the
let
block, the context object is accessed usingit
(or a custom name) - Return Value: Returns the last expression in the lambda
- Null Safety: Often used with the safe call operator (
?.
)
Let's look at a practical example that demonstrates these characteristics:
class UserService {
private val cache = mutableMapOf<String, User>()
fun getUser(id: String): User? {
return cache[id]?.let { cachedUser ->
// Using named parameter instead of 'it'
println("Cache hit for user: ${cachedUser.name}")
updateLastAccessed(cachedUser)
cachedUser // Return the user after updating
} ?: run {
// Cache miss - load from database
loadFromDatabase(id)
}
}
}
Common Use Cases for let
1. Null Safety Checks with Transformations
One of the most common and powerful uses of let
is handling nullable values while transforming them:
// Instead of
val nullableName: String? = "John"
val greeting: String
if (nullableName != null) {
greeting = "Hello, ${nullableName.uppercase()}"
} else {
greeting = "Hello, Guest"
}
// Use let
val greeting = nullableName?.let {
"Hello, ${it.uppercase()}"
} ?: "Hello, Guest"
2. Scoped Variable Usage
When you need to use a value only within a specific scope:
fun processFile(path: String) {
FileInputStream(path).let { fileStream ->
// fileStream is only accessible within this block
fileStream.use { stream ->
// Process the file
val content = stream.readBytes()
// ...process content...
}
}
// fileStream is out of scope here - preventing accidental usage
}
3. Method Chaining
let
is excellent for creating readable method chains:
fun createUser(request: UserRequest) =
validateRequest(request)
.let { validatedRequest ->
User(
name = validatedRequest.name,
email = validatedRequest.email
)
}
.let { user ->
userRepository.save(user)
}
.let { savedUser ->
UserResponse.fromUser(savedUser)
}
Comparing let with Other Scope Functions
Understanding when to use let
versus other scope functions is crucial:
let vs apply
// let: Transform the object
val userDto = user.let {
UserDto(
name = it.name,
email = it.email
)
}
// apply: Configure the object
val user = User().apply {
name = "John"
email = "[email protected]"
}
let vs run
// let: Access object as 'it', good for transformations
val length = str?.let { it.length }
// run: Access object as 'this', good for operating on object
val length = str?.run { this.length }
let vs also
// let: Transform and return new value
val processed = input.let {
processInput(it) // Returns processed result
}
// also: Perform side effect and return original
val processed = input.also {
logger.info("Processing: $it") // Returns input
}
Best Practices
1. Use Named Parameters for Clarity
When the logic inside let
is more complex, use named parameters instead of it
:
person?.let { currentPerson ->
val firstName = currentPerson.name.split(" ").first()
val lastName = currentPerson.name.split(" ").last()
formatName(firstName, lastName)
}
2. Chain let with Elvis Operator
Combine let
with the Elvis operator for elegant null handling:
fun getDisplayName(user: User?): String {
return user?.let {
when {
it.fullName.isNotBlank() -> it.fullName
it.email.isNotBlank() -> it.email
else -> it.id.toString()
}
} ?: "Unknown User"
}
3. Keep let Blocks Focused
Each let
block should have a single responsibility:
// Good: Each let has a clear purpose
user?.let { validateUser(it) }
?.let { enrichUserData(it) }
?.let { saveUser(it) }
// Avoid: Too many operations in one block
user?.let {
validateUser(it)
enrichUserData(it)
saveUser(it)
// Hard to follow the flow
}
Common Pitfalls
1. Nested let Blocks
Avoid nesting let
blocks deeply:
// Avoid this
user?.let { user ->
user.address?.let { address ->
address.city?.let { city ->
// Too much nesting
}
}
}
// Better approach
when {
user?.address?.city != null -> processCity(user.address.city)
else -> handleMissingCity()
}
2. Using let When Not Needed
Don't use let
just for the sake of using it:
// Unnecessary use of let
val name = "John"
name.let {
println(it) // Just use name directly
}
// Better
println(name)
Conclusion
The let
function is a powerful tool in Kotlin's scope function family. It excels at null safety checks, transformations, and creating readable method chains. By understanding its unique characteristics and following best practices, you can write more concise and maintainable code.
Remember that let
is most valuable when you need to:
- Transform an object into something else
- Work with nullable values
- Create a limited scope for variables
- Chain multiple operations with clear transformations
Choose let
when these are your primary goals, and consider other scope functions when different characteristics would better serve your needs.