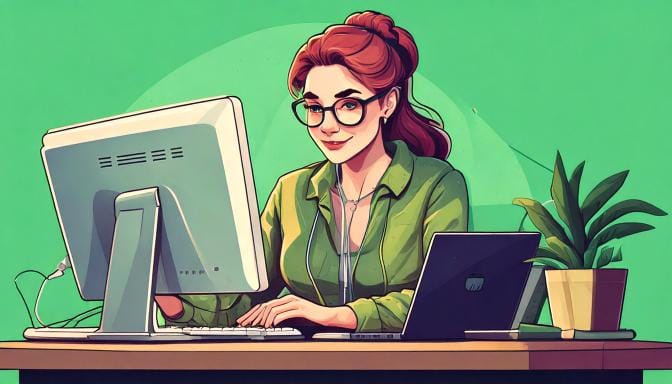
Spring Autowiring in Kotlin: A Complete Guide
Learn how to effectively use Spring's autowiring capabilities in Kotlin applications. This guide covers constructor injection, component scanning, testing strategies, and best practices for clean, maintainable code.
Dependency injection is a core concept in Spring Boot applications. This guide covers everything you need to know about autowiring in Kotlin Spring Boot applications.
Key Takeaways
- Autowiring eliminates the need for manual dependency management
- Constructor injection is preferred over field injection in Kotlin
- Use
@Autowired
sparingly - Kotlin constructors handle most cases - Component scanning automatically detects your Spring beans
Understanding Autowiring
Autowiring is Spring's mechanism for automatically injecting dependencies. In Kotlin, it's even more streamlined than in Java thanks to Kotlin's concise constructor syntax.
When working with repositories (see our comprehensive guide on Repository Pattern in Spring Boot with Kotlin), autowiring helps manage dependencies efficiently.
Constructor Injection (Recommended)
@Service
class UserService(
private val userRepository: UserRepository,
private val emailService: EmailService
) {
fun createUser(user: User) {
userRepository.save(user)
emailService.sendWelcomeEmail(user)
}
}
Spring automatically injects dependencies through the constructor. No @Autowired
annotation needed!
Field Injection (Not Recommended)
@Service
class UserService {
@Autowired
private lateinit var userRepository: UserRepository
@Autowired
private lateinit var emailService: EmailService
fun createUser(user: User) {
userRepository.save(user)
emailService.sendWelcomeEmail(user)
}
}
While this works, it's harder to test and masks dependencies.
Optional Dependencies
Sometimes you need optional dependencies. Here's how to handle them:
@Service
class NotificationService(
private val emailService: EmailService,
@Autowired(required = false)
private val smsService: SmsService? = null
) {
fun notify(user: User, message: String) {
emailService.sendEmail(user, message)
smsService?.sendSms(user, message)
}
}
Component Scanning
Spring automatically finds your components through component scanning:
@Configuration
@ComponentScan(basePackages = ["com.example.myapp"])
class AppConfig
// These will be automatically detected:
@Component
class MyComponent
@Service
class MyService
@Repository
class MyRepository
Common Pitfalls
Circular Dependencies
Avoid circular dependencies - they make your code harder to understand and can cause runtime issues:
// Don't do this:
@Service
class ServiceA(private val serviceB: ServiceB)
@Service
class ServiceB(private val serviceA: ServiceA)
Missing Component Annotations
Make sure your classes are annotated with @Component
, @Service
, @Repository
, or @Controller
:
// This won't be autowired:
class MyService(private val repository: MyRepository)
// This will work:
@Service
class MyService(private val repository: MyRepository)
Best Practices
- Use constructor injection by default
- Keep components focused and single-purpose
- Avoid field injection
- Use meaningful component names
- Consider using interfaces for better testability
Testing Autowired Components
Testing becomes straightforward with constructor injection:
@Test
fun `test user creation`() {
val mockRepository = mockk<UserRepository>()
val mockEmailService = mockk<EmailService>()
val userService = UserService(mockRepository, mockEmailService)
// Test implementation
}
Conclusion
Autowiring in Kotlin Spring Boot applications is powerful and straightforward. Stick to constructor injection, keep your components focused, and let Spring handle the dependency management for you.