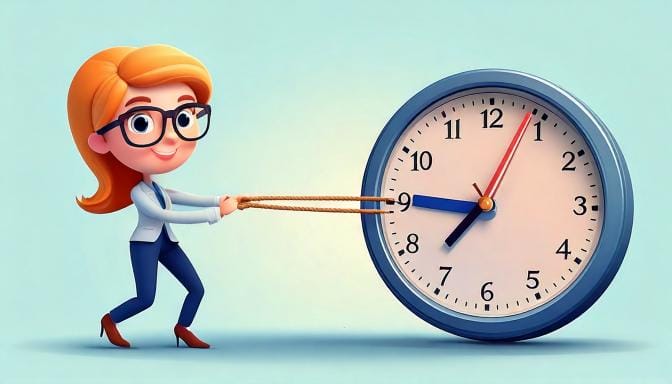
Spring Boot Scheduling with Kotlin: A Comprehensive Guide
Learn how to implement robust task scheduling in Spring Boot using Kotlin, from basic fixed-rate tasks to complex cron expressions with real-world examples.
Introduction
Task scheduling is a crucial feature in modern applications, enabling automated execution of tasks at specified intervals or times. Spring Boot provides robust scheduling capabilities that integrate seamlessly with Kotlin applications. In this guide, you'll learn how to implement various scheduling patterns in a Spring Boot application using Kotlin.
Prerequisites
To follow along with this tutorial, you'll need:
- Kotlin 1.8.0 or later
- Spring Boot 3.2.0 or later
- JDK 17 or later
- Your preferred IDE (IntelliJ IDEA recommended for Kotlin development)
- Basic knowledge of Kotlin and Spring Boot
Step 1 — Setting Up Your Project
First, create a new Spring Boot project with Kotlin. You can do this using Spring Initializer (https://start.spring.io) with the following dependencies:
dependencies {
implementation("org.springframework.boot:spring-boot-starter")
implementation("org.jetbrains.kotlin:kotlin-reflect")
implementation("org.jetbrains.kotlin:kotlin-stdlib-jdk8")
}
Enable scheduling in your application by adding the @EnableScheduling
annotation to your main application class:
import org.springframework.boot.autoconfigure.SpringBootApplication
import org.springframework.scheduling.annotation.EnableScheduling
@SpringBootApplication
@EnableScheduling
class SchedulingDemoApplication
fun main(args: Array<String>) {
runApplication<SchedulingDemoApplication>(*args)
}
Step 2 — Creating Your First Scheduled Task
Let's start with a simple scheduled task that runs at a fixed rate:
import org.springframework.scheduling.annotation.Scheduled
import org.springframework.stereotype.Component
import java.time.LocalDateTime
import org.slf4j.LoggerFactory
@Component
class ScheduledTasks {
private val logger = LoggerFactory.getLogger(ScheduledTasks::class.java)
@Scheduled(fixedRate = 5000) // Runs every 5 seconds
fun reportCurrentTime() {
logger.info("Current time is: ${LocalDateTime.now()}")
}
}
This task will run every 5 seconds, logging the current time.
Step 3 — Understanding Scheduling Patterns
Spring Boot supports several scheduling patterns. Let's explore each one:
Fixed Rate Execution
Tasks run at a specific interval, regardless of how long the task takes:
@Scheduled(fixedRate = 5000) // 5 seconds
fun fixedRateTask() {
logger.info("Fixed rate task executed")
}
Fixed Delay Execution
The next execution starts after a fixed delay from the completion of the previous execution:
@Scheduled(fixedDelay = 5000) // 5 seconds delay after completion
fun fixedDelayTask() {
logger.info("Fixed delay task executed")
}
Initial Delay
Add an initial delay before the first execution:
@Scheduled(fixedRate = 5000, initialDelay = 10000) // 10 seconds initial delay
fun delayedTask() {
logger.info("Delayed task executed")
}
Cron Expressions
Use cron expressions for more complex scheduling patterns:
@Scheduled(cron = "0 0 9 * * MON-FRI") // 9 AM on weekdays
fun workdayMorningTask() {
logger.info("Workday morning task executed")
}
Step 4 — Handling Long-Running Tasks
For long-running tasks, it's important to handle them asynchronously to prevent blocking:
import org.springframework.scheduling.annotation.Async
import org.springframework.scheduling.annotation.EnableAsync
@Configuration
@EnableAsync
class AsyncConfig {
@Bean
fun taskExecutor(): TaskExecutor {
val executor = ThreadPoolTaskExecutor()
executor.corePoolSize = 2
executor.maxPoolSize = 4
executor.queueCapacity = 10
executor.setThreadNamePrefix("AsyncTask-")
executor.initialize()
return executor
}
}
@Component
class LongRunningTasks {
private val logger = LoggerFactory.getLogger(LongRunningTasks::class.java)
@Async
@Scheduled(fixedRate = 10000)
fun longRunningTask() {
logger.info("Starting long running task")
Thread.sleep(5000) // Simulate long processing
logger.info("Completed long running task")
}
}
Step 5 — Dynamic Scheduling
Sometimes you need to change scheduling parameters dynamically. Here's how to implement dynamic scheduling:
@Component
class DynamicScheduler {
private val logger = LoggerFactory.getLogger(DynamicScheduler::class.java)
private var scheduler: ThreadPoolTaskScheduler? = null
private var future: ScheduledFuture<*>? = null
@PostConstruct
fun init() {
scheduler = ThreadPoolTaskScheduler().apply {
poolSize = 2
threadNamePrefix = "DynamicScheduler-"
initialize()
}
}
fun startScheduling(intervalInSeconds: Long) {
future?.cancel(false)
future = scheduler?.scheduleAtFixedRate(
{ performTask() },
Duration.ofSeconds(intervalInSeconds)
)
}
fun stopScheduling() {
future?.cancel(false)
}
private fun performTask() {
logger.info("Dynamic task executed at: ${LocalDateTime.now()}")
}
}
Step 6 — Error Handling
Implement proper error handling in your scheduled tasks:
@Component
class ResilientScheduledTasks {
private val logger = LoggerFactory.getLogger(ResilientScheduledTasks::class.java)
@Scheduled(fixedRate = 5000)
fun taskWithErrorHandling() {
try {
performRiskyOperation()
} catch (e: Exception) {
logger.error("Error in scheduled task: ${e.message}")
// Implement recovery logic here
}
}
private fun performRiskyOperation() {
// Your task logic here
}
}
Step 7 — Testing Scheduled Tasks
Here's how to test your scheduled tasks:
@SpringBootTest
class ScheduledTasksTest {
@SpyBean
lateinit var scheduledTasks: ScheduledTasks
@Test
fun `verify scheduled task execution`() {
verify(scheduledTasks, timeout(6000).atLeast(1))
.reportCurrentTime()
}
}
Best Practices
- Task Independence: Ensure scheduled tasks are independent and can run in parallel when needed.
- Error Handling: Always implement proper error handling to prevent task failures from affecting the application.
- Monitoring: Add appropriate logging and monitoring to track task execution and performance.
- Time Zones: Be explicit about time zones when using cron expressions:
@Scheduled(cron = "0 0 9 * * MON-FRI", zone = "Europe/London")
fun timeZoneAwareTask() {
// Task logic
}
- Resource Management: Use appropriate thread pools and consider the impact on system resources.
Conclusion
Spring Boot's scheduling capabilities provide a powerful way to automate tasks in your Kotlin applications. Whether you need simple fixed-rate execution or complex cron-based scheduling, Spring Boot has you covered. Remember to consider factors like error handling, testing, and resource management when implementing scheduled tasks in your applications.
Common Issues and Troubleshooting
Task Overlap
If your tasks are taking longer than the scheduled interval, you might want to prevent overlap:
@Scheduled(fixedRate = 5000)
@SchedulerLock(name = "preventOverlap")
fun nonOverlappingTask() {
// Task logic
}
Memory Leaks
Be careful with capturing variables in scheduled tasks:
@Component
class ScheduledTasksWithState {
private val cache = mutableMapOf<String, Any>()
@Scheduled(fixedRate = 5000)
fun cleanupTask() {
// Cleanup old entries
cache.entries.removeIf { it.value.isExpired() }
}
}
For additional help or specific use cases, consult the Spring Framework documentation.