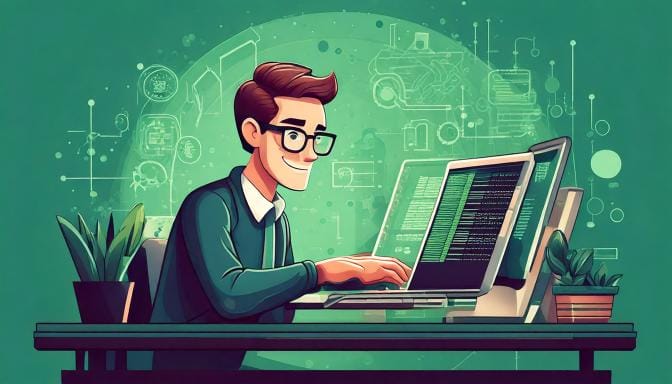
Understanding @Transactional in Spring Boot with Kotlin: A Deep Dive
Learn how @Transactional works in Spring Boot with Kotlin, from basic usage to advanced scenarios. Master isolation levels, propagation, read-only optimizations, and best practices with practical examples
Database transactions are fundamental to maintaining data integrity in your applications. Spring's @Transactional
annotation provides a powerful way to manage these transactions declaratively. In this comprehensive guide, we'll explore how to use @Transactional
effectively in your Spring Boot applications with Kotlin, understanding not just the how, but the why behind transaction management.
What Is a Transaction?
Before diving into the @Transactional
annotation, let's understand what a database transaction actually is. Think of a bank transfer: when you transfer money from one account to another, two operations need to happen:
- Deduct money from the source account
- Add money to the destination account
These operations must either both succeed or both fail. You never want a situation where money is deducted but not added to the destination account. This is where transactions come in - they ensure operations are atomic (all-or-nothing).
Basic Usage of @Transactional
Let's start with a simple example of how to use @Transactional
:
import org.springframework.transaction.annotation.Transactional
import org.springframework.stereotype.Service
@Service
class BankTransferService(
private val accountRepository: AccountRepository
) {
@Transactional
fun transferMoney(fromAccountId: Long, toAccountId: Long, amount: BigDecimal) {
val fromAccount = accountRepository.findById(fromAccountId)
.orElseThrow { AccountNotFoundException(fromAccountId) }
val toAccount = accountRepository.findById(toAccountId)
.orElseThrow { AccountNotFoundException(toAccountId) }
fromAccount.balance = fromAccount.balance.subtract(amount)
toAccount.balance = toAccount.balance.add(amount)
accountRepository.save(fromAccount)
accountRepository.save(toAccount)
}
}
In this example, @Transactional
ensures that both account updates succeed or both fail. If any exception occurs during the process, both accounts will be restored to their original state.
Transaction Propagation
One of the most important aspects of @Transactional
is understanding propagation. Propagation defines how transactions should behave when one transactional method calls another. Spring provides several propagation options:
@Service
class OrderService(
private val paymentService: PaymentService,
private val inventoryService: InventoryService
) {
// This creates a new transaction
@Transactional(propagation = Propagation.REQUIRED)
fun processOrder(order: Order) {
// This will run in the same transaction as processOrder
paymentService.processPayment(order)
// This will create a new independent transaction
inventoryService.updateInventory(order)
}
}
@Service
class PaymentService {
// Uses existing transaction if present, otherwise creates new one
@Transactional(propagation = Propagation.REQUIRED)
fun processPayment(order: Order) {
// Payment processing logic
}
}
@Service
class InventoryService {
// Always creates a new transaction
@Transactional(propagation = Propagation.REQUIRES_NEW)
fun updateInventory(order: Order) {
// Inventory update logic
}
}
Let's understand the common propagation types:
REQUIRED
(Default): Use current transaction, create new one if none existsREQUIRES_NEW
: Always create a new transactionSUPPORTS
: Use current transaction if it exists, otherwise non-transactionalMANDATORY
: Must run within existing transaction, throw exception if none existsNEVER
: Must run without transaction, throw exception if transaction existsNOT_SUPPORTED
: Suspend current transaction if it exists
Transaction Isolation Levels
Transaction isolation levels determine how data modified by one transaction is visible to other concurrent transactions. Understanding these levels is crucial for maintaining data consistency while maximizing performance. Let's explore each type of potential data inconsistency and how different isolation levels prevent them.
Understanding Read Phenomena
Dirty Reads
A dirty read occurs when one transaction reads data that another transaction has modified but hasn't yet committed. Think of it like reading a bank account balance while a transfer is in progress:
// Transaction 1: Transfer money
@Transactional
fun transferMoney(accountId: Long, amount: BigDecimal) {
val account = accountRepository.findById(accountId).get()
account.balance = account.balance.subtract(amount)
accountRepository.save(account)
// Imagine the transaction hasn't committed yet
// Perhaps some validation is still happening
}
// Transaction 2: Read balance (with READ_UNCOMMITTED)
@Transactional(isolation = Isolation.READ_UNCOMMITTED)
fun getBalance(accountId: Long): BigDecimal {
val account = accountRepository.findById(accountId).get()
return account.balance // Could read the intermediate, uncommitted balance!
}
In this scenario, the second transaction might see a balance that never actually existed if the first transaction rolls back.
Non-repeatable Reads
A non-repeatable read happens when a transaction reads the same data twice and gets different values each time because another transaction modified the data between the reads. Here's an example:
// Transaction 1: Long-running report
@Transactional(isolation = Isolation.READ_COMMITTED)
fun generateProductReport() {
val product = productRepository.findById(1).get()
val initialPrice = product.price
// Some time-consuming operations happen here...
Thread.sleep(1000) // Simulating complex calculations
val sameProduct = productRepository.findById(1).get()
val currentPrice = sameProduct.price
// initialPrice might be different from currentPrice!
// Another transaction could have changed it in between
}
// Transaction 2: Price update
@Transactional
fun updatePrice(productId: Long, newPrice: BigDecimal) {
val product = productRepository.findById(productId).get()
product.price = newPrice
productRepository.save(product)
}
Phantom Reads
Phantom reads occur when a transaction reads a collection of rows twice, and in between, another transaction adds or removes rows that match the collection's search condition. Here's an example:
// Transaction 1: Report on products in a price range
@Transactional(isolation = Isolation.REPEATABLE_READ)
fun generatePriceRangeReport(minPrice: BigDecimal, maxPrice: BigDecimal) {
// First count
val initialCount = productRepository
.countByPriceBetween(minPrice, maxPrice)
// Some processing time...
Thread.sleep(1000)
// Second count
val currentCount = productRepository
.countByPriceBetween(minPrice, maxPrice)
// currentCount might be different!
// New products could have been added in the price range
}
// Transaction 2: Add new product
@Transactional
fun addProduct(product: Product) {
productRepository.save(product)
}
Choosing the Right Isolation Level
Let's look at each isolation level in detail:
@Service
class ProductService {
// Lowest isolation, highest performance
// But allows dirty reads, non-repeatable reads, and phantom reads
@Transactional(isolation = Isolation.READ_UNCOMMITTED)
fun getProductPrice(productId: Long): BigDecimal {
return productRepository.findById(productId).get().price
}
// Prevents dirty reads
// Still allows non-repeatable reads and phantom reads
@Transactional(isolation = Isolation.READ_COMMITTED)
fun updateProductPrice(productId: Long, newPrice: BigDecimal) {
val product = productRepository.findById(productId)
.orElseThrow { ProductNotFoundException(productId) }
product.price = newPrice
productRepository.save(product)
}
// Prevents dirty and non-repeatable reads
// Still allows phantom reads
@Transactional(isolation = Isolation.REPEATABLE_READ)
fun calculateTotalInventoryValue(): BigDecimal {
return productRepository.findAll()
.sumOf { it.price * it.quantity }
}
// Highest isolation, lowest performance
// Prevents all read phenomena
@Transactional(isolation = Isolation.SERIALIZABLE)
fun generateCompleteInventoryReport(): InventoryReport {
// Complex report generation logic
// No other transactions can modify the inventory
// while this runs
}
}
Here's a summary of what each isolation level prevents:
READ_UNCOMMITTED
:- Allows all read phenomena
- Highest performance
- Suitable for scenarios where absolute accuracy isn't required (e.g., rough statistics)
READ_COMMITTED
:- Prevents dirty reads
- Allows non-repeatable reads and phantom reads
- Good balance for most applications
- Default in many databases (e.g., PostgreSQL)
REPEATABLE_READ
:- Prevents dirty reads and non-repeatable reads
- Allows phantom reads
- Good for reports that need consistent data views
- Default in some databases (e.g., MySQL)
SERIALIZABLE
:- Prevents all read phenomena
- Lowest performance (can cause significant blocking)
- Use only when absolute data consistency is required
When choosing an isolation level, consider these factors:
- How critical is data consistency for your use case?
- What is the concurrent load on your system?
- What kind of read phenomena can your application tolerate?
- What is the performance impact acceptable for your application?
For most applications, READ_COMMITTED
provides a good balance between consistency and performance. Use higher isolation levels only when you have specific requirements that justify the performance impact.
Read-Only Transactions
When you're only reading data, you can optimize performance by marking the transaction as read-only. Understanding how this optimization works internally helps us use it more effectively.
How Read-Only Transactions Work
When a regular transaction starts, the database and Hibernate (JPA implementation) prepare for potential data modifications by:
- Creating transaction logs (redo/undo logs)
- Maintaining version information for optimistic locking
- Performing dirty checking on entities
- Keeping first-level cache synchronized
However, when you mark a transaction as read-only, Spring and Hibernate can make several optimizations:
@Service
class ReportService {
@Transactional(readOnly = true)
fun generateDailySalesReport(date: LocalDate): SalesReport {
// 1. No transaction logs created
// 2. No entity snapshots maintained
// 3. Hibernate dirty checking disabled
val sales = salesRepository.findByDate(date)
return SalesReport.fromSales(sales)
}
}
Let's examine these optimizations in detail:
Database-Level Optimizations
At the database level, read-only transactions allow the database to:
- Skip write-ahead logging (WAL)
- Avoid maintaining undo segments
- Potentially use different buffer pool strategies
Here's a practical example:
@Service
class ProductCatalogService(
private val productRepository: ProductRepository
) {
// Regular transaction - database prepares for potential writes
@Transactional
fun getProductDetails(id: Long): ProductDetails {
val product = productRepository.findById(id).orElseThrow()
return ProductDetails(product)
}
// Read-only transaction - database can optimize for reading
@Transactional(readOnly = true)
fun getProductDetailsReadOnly(id: Long): ProductDetails {
val product = productRepository.findById(id).orElseThrow()
return ProductDetails(product)
}
}
Hibernate-Level Optimizations
Hibernate makes several important optimizations for read-only transactions:
- Disabled Dirty Checking: Hibernate doesn't need to create snapshots of entities or check for changes:
@Service
class InventoryService(
private val sessionFactory: SessionFactory
) {
@Transactional(readOnly = true)
fun getLargeInventoryReport(): InventoryReport {
// In a regular transaction, Hibernate would:
// 1. Create snapshots of all loaded entities
// 2. Compare entities with snapshots at commit
// 3. Maintain version information
// With readOnly=true, these operations are skipped
// Significantly reducing memory usage for large result sets
val inventory = sessionFactory.currentSession
.createQuery("FROM Product p JOIN FETCH p.category")
.list()
return InventoryReport(inventory)
}
}
- Flush Mode Optimization: Hibernate can skip unnecessary flush operations:
@Service
class OrderAnalyticsService(
private val orderRepository: OrderRepository
) {
@Transactional(readOnly = true)
fun analyzeOrders(): OrderAnalytics {
// In a regular transaction, Hibernate might flush
// the session during complex queries to ensure consistency
// With readOnly=true, these flushes are avoided
// as we know the data won't be modified
val totalOrders = orderRepository.count()
val averageOrderValue = orderRepository.getAverageOrderValue()
val topProducts = orderRepository.findTopSellingProducts()
return OrderAnalytics(totalOrders, averageOrderValue, topProducts)
}
}
When to Use Read-Only Transactions
Read-only transactions are particularly beneficial in these scenarios:
@Service
class ReportingService(
private val orderRepository: OrderRepository,
private val productRepository: ProductRepository
) {
// Perfect for reporting queries
@Transactional(readOnly = true)
fun generateMonthlyReport(): MonthlyReport {
val orders = orderRepository.findAllByMonth(LocalDate.now().month)
return MonthlyReport.create(orders)
}
// Great for large result sets
@Transactional(readOnly = true)
fun exportProductCatalog(): ProductCatalog {
val products = productRepository.findAll()
.map { ProductInfo(it) }
return ProductCatalog(products)
}
// Ideal for dashboard queries
@Transactional(readOnly = true)
fun getDashboardMetrics(): DashboardMetrics {
return DashboardMetrics(
totalOrders = orderRepository.count(),
averageOrderValue = orderRepository.getAverageOrderValue(),
topProducts = productRepository.findTopSellingProducts(limit = 10)
)
}
}
Performance Impact
The performance benefits of read-only transactions become more noticeable when:
- Dealing with large result sets (reduced memory usage)
- Having high concurrency (reduced lock contention)
- Running complex queries (optimized query plans)
- Processing data in bulk (no overhead for change tracking)
Here's an example showing how to measure the difference:
@Service
class PerformanceTestService(
private val productRepository: ProductRepository
) {
// Regular transaction
@Transactional
fun loadProductsRegular(): List<Product> {
val startTime = System.currentTimeMillis()
val products = productRepository.findAll()
logger.info("Regular transaction took: ${System.currentTimeMillis() - startTime}ms")
return products
}
// Read-only transaction
@Transactional(readOnly = true)
fun loadProductsReadOnly(): List<Product> {
val startTime = System.currentTimeMillis()
val products = productRepository.findAll()
logger.info("Read-only transaction took: ${System.currentTimeMillis() - startTime}ms")
return products
}
}
Remember that while read-only transactions provide these optimizations, they also enforce constraints:
- You cannot modify any entities within the transaction
- Any attempt to modify data will result in an exception
- The optimizations are primarily beneficial for larger datasets or high-concurrency scenarios
For small, simple queries, the performance difference might be negligible, but for large-scale operations, the benefits can be substantial.
Exception Handling and Rollback Rules
By default, @Transactional
rolls back on unchecked exceptions but commits on checked exceptions. You can customize this behavior:
@Service
class OrderProcessingService {
// Rolls back on any Exception
@Transactional(rollbackFor = [Exception::class])
fun processOrder(order: Order) {
// Processing logic
}
// Won't rollback on BusinessException
@Transactional(noRollbackFor = [BusinessException::class])
fun updateOrderStatus(orderId: Long, status: OrderStatus) {
// Update logic
}
}
Common Pitfalls and Best Practices
1. Self-Invocation Problem
One common pitfall is calling a @Transactional
method from within the same class:
@Service
class UserService {
// This transaction won't work when called from createUser!
@Transactional
fun updateUserProfile(user: User) {
// Update logic
}
fun createUser(user: User) {
// This calls the method directly, bypassing Spring's proxy
updateUserProfile(user) // Transaction won't be applied!
}
}
The solution is to use self-injection or separate the methods into different services:
@Service
class UserService(
private val self: UserService
) {
@Transactional
fun updateUserProfile(user: User) {
// Update logic
}
fun createUser(user: User) {
// This works because it goes through Spring's proxy
self.updateUserProfile(user)
}
}
2. Transaction Boundary Placement
When implementing repositories in Spring Boot (see our comprehensive guide on the Repository Pattern in Spring Boot with Kotlin), it's crucial to understand how transactions interact with your data access layer. The Repository pattern provides a clean abstraction for data access, but proper transaction management ensures data consistency.
Place transaction boundaries appropriately to include all related operations:
@Service
class OrderService(
private val orderRepository: OrderRepository,
private val emailService: EmailService
) {
@Transactional
fun createOrder(order: Order) {
orderRepository.save(order)
// Bad: Email sending shouldn't be part of the transaction
emailService.sendOrderConfirmation(order)
}
// Better approach
fun processOrderWithEmail(order: Order) {
// Transaction only around database operations
createOrder(order)
// Email sending outside transaction
emailService.sendOrderConfirmation(order)
}
}
3. Using Constructor Injection
Always use constructor injection with @Transactional
services:
// Good: Constructor injection
@Service
class OrderService(
private val orderRepository: OrderRepository,
private val paymentService: PaymentService
)
// Bad: Field injection
@Service
class OrderService {
@Autowired
private lateinit var orderRepository: OrderRepository
@Autowired
private lateinit var paymentService: PaymentService
}
Advanced Use Cases
1. Programmatic Transaction Management
Sometimes you need more control over transactions than annotations provide:
@Service
class ComplexOrderService(
private val transactionTemplate: TransactionTemplate,
private val orderRepository: OrderRepository
) {
fun processComplexOrder(order: Order) {
val result = transactionTemplate.execute { status ->
try {
// Complex transaction logic
orderRepository.save(order)
true // Commit
} catch (e: Exception) {
status.setRollbackOnly()
false // Rollback
}
}
}
}
2. Transaction Events
Spring provides transaction-bound events:
@Service
class OrderService {
@Transactional
fun createOrder(order: Order): Order {
val savedOrder = orderRepository.save(order)
// This event will be published only if transaction commits
applicationEventPublisher.publishEvent(
OrderCreatedEvent(savedOrder)
)
return savedOrder
}
}
@Component
class OrderEventListener {
@TransactionalEventListener(
phase = TransactionPhase.AFTER_COMMIT
)
fun handleOrderCreated(event: OrderCreatedEvent) {
// Handle the event after transaction commits
}
}
Testing Transactional Code
Testing transactional code requires special attention:
@SpringBootTest
class OrderServiceTest {
@Autowired
private lateinit var orderService: OrderService
@Autowired
private lateinit var orderRepository: OrderRepository
@Test
@Transactional // Roll back after test
fun `should process order successfully`() {
// Test logic
}
@Test
@Commit // Commit changes after test
fun `should persist order changes`() {
// Test logic
}
}
Performance Considerations
- Keep transactions as short as possible
- Use read-only transactions when appropriate
- Choose the right isolation level for your use case
- Be careful with long-running transactions
- Consider using batch operations for large datasets
Conclusion
Understanding @Transactional
is crucial for building robust Spring Boot applications. By mastering its features and avoiding common pitfalls, you can ensure your application maintains data consistency while performing efficiently.
Remember these key points:
- Use transactions to maintain data consistency
- Choose appropriate propagation and isolation levels
- Keep transactions focused and short-lived
- Be aware of common pitfalls like self-invocation
- Test transactional code thoroughly